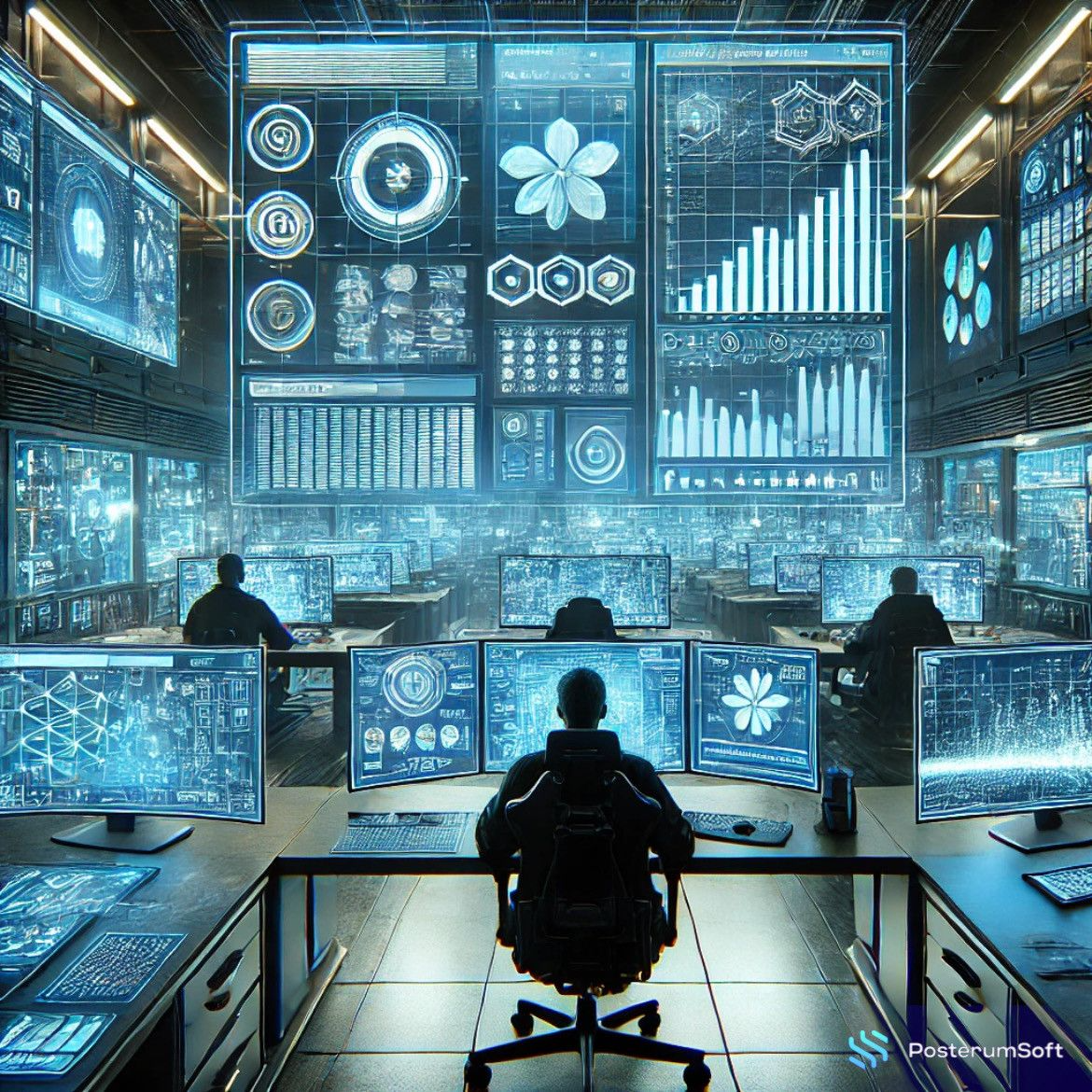
How to Optimize Game Development Processes with Administrative Panels
Discover how administrative panels can enhance game development efficiency, improve team collaboration, and reduce costs...
Sep 19, 2024
In today’s world of web development, asynchronous programming is essential for building high-performance, responsive, and scalable applications. Vue.js simplifies handling asynchronous operations with `async` and `await`, allowing developers to write more readable and maintainable code. This article outlines the best practices and advanced techniques to help you leverage Vue.js's asynchronous capabilities effectively.
The `async` and `await` keywords allow you to write asynchronous code that looks synchronous, making it easier to read and debug. With `async`, you define an asynchronous function, and `await` pauses the function execution until the awaited operation is completed, without blocking the thread.
export default {
async mounted() {
await this.loadData();
},
methods: {
async loadData() {
try {
const response = await fetch('/api/data');
const data = await response.json();
console.log(data);
} catch (error) {
console.error('Error loading data:', error);
}
}
}
};
Blocking operations, like using `then()` or `catch()`, can slow down your app and reduce its responsiveness. Instead, always use `await` to handle asynchronous operations without blocking the UI.
// Bad practice
fetch('/api/data').then(response => response.json()).then(data => {
console.log(data);
});
// Best practice
const data = await fetch('/api/data').then(response => response.json());
It’s important to handle exceptions in your async functions with `try-catch` blocks to ensure any potential errors are properly addressed.
async loadData() {
try {
const response = await fetch('/api/data');
const data = await response.json();
return data;
} catch (error) {
console.error('Error fetching data:', error);
}
}
When you need to perform several asynchronous operations in sequence, use loops with `await` to process them.
async fetchMultipleData() {
const urls = ['/api/data1', '/api/data2', '/api/data3'];
for (const url of urls) {
const response = await fetch(url);
const data = await response.json();
console.log(data);
}
}
In cases where you need to handle a stream of asynchronous data, `for-await-of` allows you to iterate over asynchronous data sources.
async function* fetchDataStream() {
const urls = ['/api/data1', '/api/data2', '/api/data3'];
for (const url of urls) {
const response = await fetch(url);
const data = await response.json();
yield data;
}
}
async function processStream() {
for await (const data of fetchDataStream()) {
console.log(data);
}
}
If you have multiple asynchronous operations that don’t depend on each other, use `Promise.all` to execute them in parallel, significantly reducing the total execution time.
async loadDataInParallel() {
const [data1, data2, data3] = await Promise.all([
fetch('/api/data1').then(res => res.json()),
fetch('/api/data2').then(res => res.json()),
fetch('/api/data3').then(res => res.json())
]);
console.log(data1, data2, data3);
}
In Vuex (or Pinia), asynchronous actions play a critical role in state management.
const store = createStore({
actions: {
async fetchData({ commit }) {
const data = await fetch('/api/data').then(res => res.json());
commit('setData', data);
}
}
});
Async file operations can significantly improve performance when handling I/O-bound tasks, such as reading or writing files.
methods: {
async readFile(file) {
const reader = new FileReader();
return new Promise((resolve, reject) => {
reader.onload = () => resolve(reader.result);
reader.onerror = reject;
reader.readAsText(file);
});
}
}
Incorporating `async` and `await` into your Vue.js applications can greatly simplify asynchronous programming, making your code cleaner, more efficient, and easier to maintain. By following these best practices and applying advanced techniques, you can create scalable, high-performance applications that offer an enhanced user experience. Vue.js provides powerful tools for developers, and mastering its asynchronous capabilities is key to building responsive web apps.
Contact PosterumSoft and find out how we can help you optimize your frontend development and scale your business.
Follow us on LinkedIn: linkedin.com
Follow us on X: x.com
Follow us on Medium: medium.com
Check us out on Upwork: upwork.com
Discover how administrative panels can enhance game development efficiency, improve team collaboration, and reduce costs...
Master advanced techniques in Vue.js to improve performance, security, and scalability for your applications...