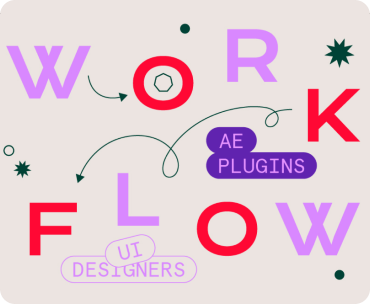
Choosing the Right IDE for JavaScript Development: Exploring the Differences
As a JavaScript developer, having the right Integrated Development Environment (IDE) is crucial for efficient coding, debugging, and project managemen...
Jul 6, 2023
Introduction: As a web developer, JavaScript is your go-to language for creating dynamic and interactive websites. Understanding JavaScript’s data types is crucial, as they form the foundation of any JavaScript application. In this article, we’ll dive into the various data types in JavaScript and explore their unique characteristics through practical examples. Whether you’re a beginner or an experienced developer, this guide will equip you with the knowledge to wield JavaScript’s power effectively.
JavaScript has six primitive data types: number, string, boolean, null, undefined, and symbol. Let's explore each type with examples:
number: Represents numeric values, such as whole numbers or decimals. For instance:
let age = 28;
let price = 9.99;
string: Denotes textual data and is enclosed within single or double quotes. Examples:
let name = 'John Doe';
let message = "Hello, world!";
boolean: Represents logical values true or false. They are often used in conditionals. Examples:
let isActive = true;
let isLoggedOut = false;
null and undefined: Indicate the absence of a value. Examples:
let data = null; // explicitly assigned absence of an object
let address; // undefined, uninitialized variable
symbol: Used as unique identifiers for object properties. Example:
const KEY = Symbol('my key');
let obj = {
[KEY]: 'value'
};
JavaScript provides two composite data types: object and array. These types can hold multiple values and are mutable. Let's explore them:
object: A collection of key-value pairs. Examples:
let person = {
name: 'John',
age: 25,
address: '123 Main St'
};
console.log(person.name); // Output: John
array: An ordered list of values. Examples:
let numbers = [1, 2, 3, 4, 5];
console.log(numbers[0]); // Output: 1
let fruits = ['apple', 'banana', 'orange'];
console.log(fruits.length); // Output: 3
JavaScript also has special data types worth mentioning:
function: Functions are objects in JavaScript, enabling code reuse and functional programming. Example:
function add(a, b) {
return a + b;
}
let result = add(3, 5);
console.log(result); // Output: 8
date: The Date object allows working with dates and times. Example:
let currentDate = new Date();
console.log(currentDate); // Output: current date and time
Conclusion: By understanding JavaScript’s data types and their practical usage through examples, you’re better equipped to write efficient and bug-free code. Whether you’re working with numbers, text, collections, or functions, JavaScript’s data types provide the versatility to handle various scenarios. Embrace this knowledge and leverage the full potential of JavaScript in your web development journey. Happy coding!
Note: This article assumes a basic familiarity with JavaScript programming concepts and syntax.
As a JavaScript developer, having the right Integrated Development Environment (IDE) is crucial for efficient coding, debugging, and project managemen...
JavaScript is an indispensable language in the realm of web development. It empowers developers to create dynamic and interactive websites by enhancin...