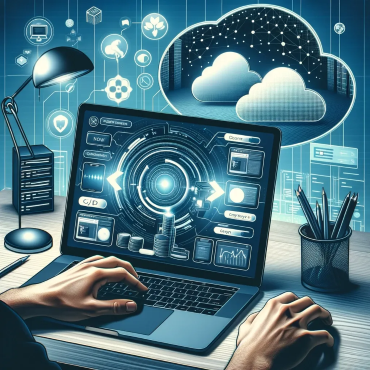
Browser architecture
For a long time, I believed that knowledge of browser architecture was not necessary to write web applications. Many people learn HTML for layout in s...
Aug 4, 2023
Writing clean and maintainable code is a fundamental skill for every developer, and adhering to proper naming conventions plays a crucial role in achieving this goal. In this article, we will explore ten JavaScript naming conventions that can significantly enhance code readability and improve collaboration among team members.
JavaScript variable names are case-sensitive, meaning that lowercase and uppercase letters are distinct. To avoid confusion and ensure consistency, adopt camel case for all variable names. Use meaningful and descriptive names that reflect the purpose of the variable. For instance:
// case-sensetive
let PetName = 'Hachico';
let petName = 'Toto';
let PETNAME = 'Baxter';
console.log(PetName); // "Hachico"
console.log(petName); // "Toto"
console.log(PETNAME); // "Baxter"
// Bad
let d = 'Hachiko';
// Bad
let name = 'Hachiko';
// Good
let petName = 'Hachiko';
When working with Boolean variables, consider using prefixes like "is" or "has" to indicate their purpose. For example:
// Bad
let dark = false;
// Good
let isDark = false;
// bad
let ideal = true;
// good
let areIdeal = true;
// bad
let owner = true;
// good
let hasOwner = true;
JavaScript function names should also be written in camel case, and they should be descriptive. Using nouns and verbs as prefixes helps convey the function's purpose effectively. For example:
// Bad
function name(petName, ownerName) {
return `${dogName} ${ownerName}`;
}
// Good
function getName(petName, ownerName) {
return `${petName} ${ownerName}`;
}
Constants in JavaScript are non-changing variables and should be written in uppercase. Adopt the UPPER_SNAKE_CASE convention when declaring constants with multiple words.
// Good
const LEG = 4;
const TAIL = 1;
const MOVABLE = LEG + TAIL;
Class names should be descriptive, highlighting the class's capabilities. Use Pascal case (capitalizing the first letter of each word) for class names.
// Good
class PetCartoon {
constructor(petName, ownerName) {
this.petName = petName;
this.ownerName = ownerName;
}
}
When working with components, particularly in front-end frameworks like React, treat them like classes and use Pascal case for component names. This practice helps differentiate them from native HTML elements and improves code organization.
// Good
function PetCartoon(roles) {
return (
<div>
<span> Pet Name: {roles.petName} </span>
<span> Owner Name: {roles.ownerName} </span>
</div>
);
}
Naming conventions for methods are similar to functions. Use camel case and prefix the method name with verbs to indicate their action or operation.
class PetCartoon {
constructor(petName, ownerName) {
this.petName = petName;
this.ownerName = ownerName;
}
getName() {
return `${this.petName} ${this.ownerName}`;
}
}
let cartoon= new PetCartoon('Hachiko', ' Hidesaburō');
console.log(cartoon.getName());
// Output: "Hachiko Hidesaburō"
To denote private functions in JavaScript, add an underscore as a prefix to the function name.
class PetCartoon {
constructor(petName, ownerName) {
this.petName = petName;
this.ownerName = ownerName;
this.name = this._toonName(petName, ownerName);
}
_toonName(petName, ownerName) {
return `${petName} ${ownerName}`;
}
}
let cartoon= new PetCartoon('Hachiko', ' Hidesaburō');
// Good
let name = cartoon.name;
console.log(name);
// Output: "Hachiko Hidesaburō"
// Avoid using private function directly
// Bad
name =cartoon._toonName(cartoon.petName, cartoon.ownerName);
console.log(name);
// Output: "Hachiko Hidesaburō"
For global JavaScript variables, consider using camel case for mutable variables and uppercase for immutable ones.
// Good (Mutable global variable)
var maxAttempts = 3;
// Good (Immutable global variable)
const PI = 3.14159;
In file names, favor lowercase letters to ensure compatibility across different web servers, as some are case-sensitive while others are not.
// Good
// dogcartoon.js
Conclusion:
By consistently following these JavaScript naming conventions, your code will become more organized, maintainable, and easily understandable for both yourself and other developers. Implementing these practices will not only make your codebase more professional but also facilitate seamless collaboration and code integration among team members. Happy coding!
For a long time, I believed that knowledge of browser architecture was not necessary to write web applications. Many people learn HTML for layout in s...
JavaScript is a versatile programming language that plays a crucial role in web development. One of the fundamental concepts in JavaScript is variable...