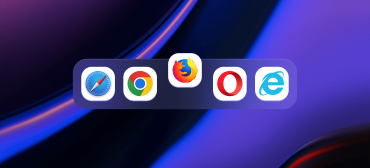
10 Essential JavaScript Naming Conventions Every Developer Should Master
Writing clean and maintainable code is a fundamental skill for every developer, and adhering to proper naming conventions plays a crucial role in achi...
Aug 3, 2023
JavaScript is a versatile programming language that plays a crucial role in web development. One of the fundamental concepts in JavaScript is variables. Variables act as containers for storing data, and they come in different types, each serving a specific purpose. In this article, we will explore the different types of JavaScript variables and understand their differences through practical examples.
The var keyword was the original way to declare variables in JavaScript. However, with the introduction of ES6 (ECMAScript 2015), let and const were introduced to address some of the issues associated with var. Despite its drawbacks, var is still used in legacy code and has a function scope.
Example:
function exampleVar() {
if (true) {
var x = 10;
}
console.log(x); // Output: 10
}
exampleVar();
console.log(x); // Output: 10 (if `var x` is declared in the global scope)let - Block-Scoped Variable
Introduced in ES6, the let keyword allows you to declare variables with block-level scope. Unlike var, let variables are confined within the nearest enclosing block (e.g., loops or conditionals) in which they are defined.
Example:
function exampleLet() {
if (true) {
let y = 5;
console.log(y); // Output: 5
}
// console.log(y); // Error: y is not defined (as it is not accessible outside the block)
}
exampleLet();
Similarly introduced in ES6, the const keyword allows you to declare constants that cannot be reassigned after initialization. This creates immutable variables, providing the added benefit of preventing unintended reassignments.
Example:
function exampleConst() {
const z = 2;
// z = 4; // Error: Assignment to constant variable
console.log(z); // Output: 2
}
exampleConst();
JavaScript has the concept of "hoisting," which allows variables declared with var to be moved to the top of their scope during the compilation phase. This means you can access a variable before it is declared, but its value will be undefined.
Example:
function exampleHoisting() {
console.log(a); // Output: undefined
var a = 7;
console.log(a); // Output: 7
}
exampleHoisting();
However, let and const variables are not hoisted, and accessing them before declaration will result in a ReferenceError.
Understanding the different types of JavaScript variables is essential for writing clean and efficient code. The var keyword has function scope, while let and const have block scope. Additionally, let allows reassignment, while const creates immutable variables. With ES6 being widely supported, it is recommended to use let and const for better code readability and maintainability, while var should generally be avoided unless necessary.
Remember to choose the appropriate variable type based on your specific needs, and always strive to write clear and concise JavaScript code for a more enjoyable development experience.
Writing clean and maintainable code is a fundamental skill for every developer, and adhering to proper naming conventions plays a crucial role in achi...
The developer console in web browsers is a powerful tool that can greatly aid in both debugging and performance optimization of web applications...